Product docs and API reference are now on Akamai TechDocs.
Search product docs.
Search for “” in product docs.
Search API reference.
Search for “” in API reference.
Search Results
 results matching 
 results
No Results
Filters
Deploy Spring Boot Applications with an NGINX Reverse Proxy
Traducciones al EspañolEstamos traduciendo nuestros guías y tutoriales al Español. Es posible que usted esté viendo una traducción generada automáticamente. Estamos trabajando con traductores profesionales para verificar las traducciones de nuestro sitio web. Este proyecto es un trabajo en curso.


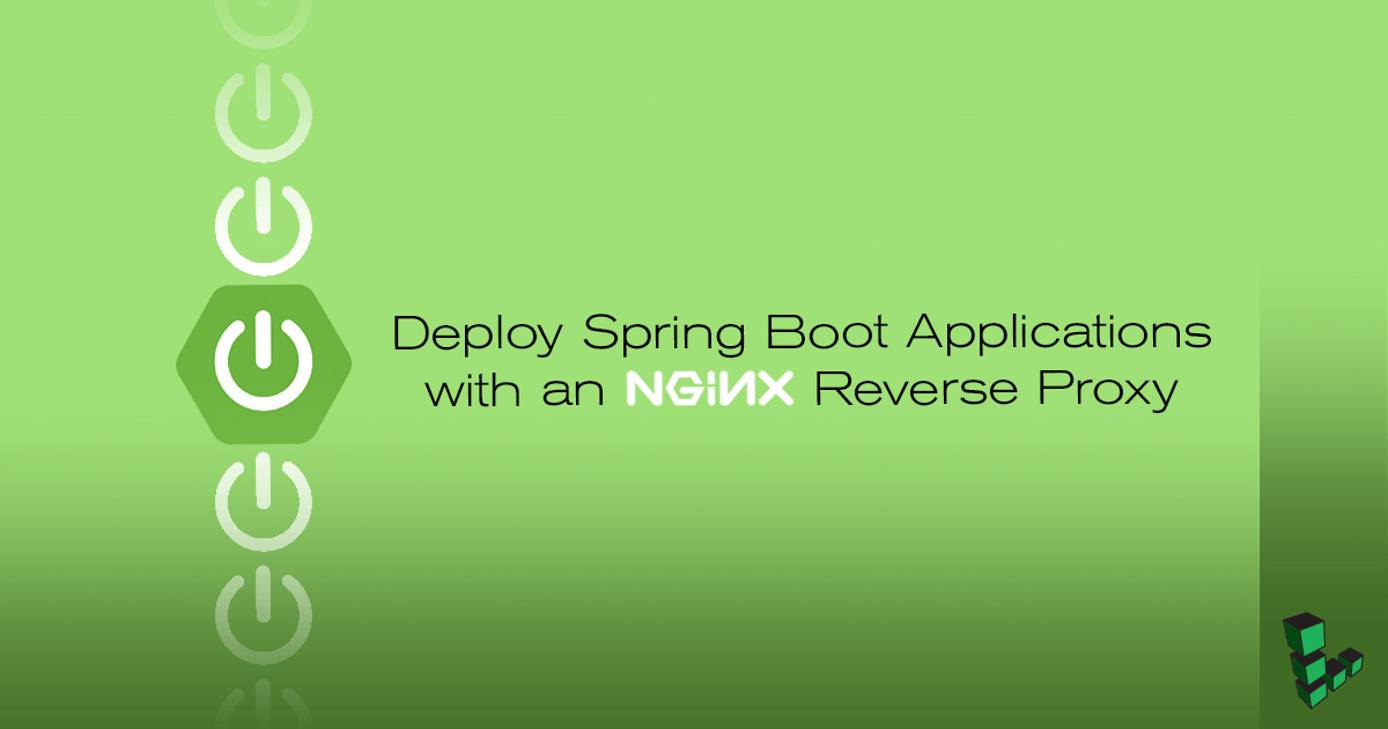
What is Spring Boot?
Spring Boot enables quick development of the Spring Framework by taking care of default configurations and allowing Java developers to focus on rapid prototyping. This guide shows how to create a simple Spring Boot application which is then exposed through an NGINX reverse proxy.
Before You Begin
You will need a Linode with both Java 8 and NGINX. If these are already installed on your Linode, skip to the next section.
Install Java JDK 8
Install
software-properties-common
:sudo apt install software-properties-common
Add the Oracle PPA repository:
sudo apt-add-repository ppa:webupd8team/java
Update your system:
sudo apt update
Install the Oracle JDK. To install the Java 9 JDK, change
java8
tojava9
in the command:sudo apt install oracle-java8-installer
Check your Java version:
java -version
Install NGINX
These steps install NGINX Mainline on Ubuntu from NGINX Inc’s official repository. For other distributions, see the NGINX admin guide. For information on configuring NGINX for production environments, see our Getting Started with NGINX series.
Open
/etc/apt/sources.list
in a text editor and add the following line to the bottom. ReplaceCODENAME
in this example with the codename of your Ubuntu release. For example, for Ubuntu 18.04, named Bionic Beaver, insertbionic
in place ofCODENAME
below:- File: /etc/apt/sources.list
1
deb http://nginx.org/packages/mainline/ubuntu/ CODENAME nginx
Import the repository’s package signing key and add it to
apt
:sudo wget http://nginx.org/keys/nginx_signing.key sudo apt-key add nginx_signing.key
Install NGINX:
sudo apt update sudo apt install nginx
Ensure NGINX is running and enabled to start automatically on reboot:
sudo systemctl start nginx sudo systemctl enable nginx
Install Spring Boot CLI
The Spring Boot CLI makes creating a scaffold for a project much easier. SDKMAN! is a tool that simplifies installation of the Spring CLI and build tools such as Gradle or Maven. Using the Spring Boot CLI, a new project can be created directly from the command line.
Install dependencies for SDKMAN!:
sudo apt install unzip zip
Install SDKMAN!:
curl -s https://get.sdkman.io | bash
Follow the instructions printed on the console:
source "/home/username/.sdkman/bin/sdkman-init.sh"
Verify SDKMAN! is installed:
sdk help
Install Spring CLI:
sdk install springboot
Verify the installation:
spring version
Install Gradle:
sdk install gradle 4.5.1
Downloading: gradle 4.5.1 In progress... ######################################################################## 100.0% Installing: gradle 4.5.1 Done installing!
Build a jar File
There are many build tools available. The Spring Boot CLI uses Maven by default but this guide will use Gradle instead. See this comparison for a discussion about the differences between Maven and Gradle.
Create a new project with the Spring Boot CLI. This creates a new directory called
hello-world
with a project scaffold.spring init --build=gradle --dependencies=web --name=hello hello-world
Note To see a full list of possible parameters for the Spring Boot CLI, run:
spring init --list
Navigate into the project directory. This example creates an endpoint to return “Hello world” in a Spring application. Add two additional imports and a new class for this mapping.
- File: ~/hello-world/src/main/java/com/example/helloworld/HelloApplication.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
package com.example.helloworld; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.web.bind.annotation.RestController; import org.springframework.web.bind.annotation.RequestMapping; @SpringBootApplication public class HelloApplication { public static void main(String[] args) { SpringApplication.run(HelloApplication.class, args); } } @RestController class Hello { @RequestMapping("/") String index() { return "Hello world"; } }
Build the application. This creates a new directory called
build
in the project../gradlew build
Run the application embedded with the Tomcat Server. The application will run on a Tomcat servlet on
localhost:8080
. PressCtrl+C
to stop.java -jar build/libs/hello-world-0.0.1-SNAPSHOT.jar
The application can run in-place without building a jar file first.
gradle bootRun
Test that the application is running correctly on
localhost
:curl localhost:8080
Hello world
Stop the Tomcat server with
CTRL+C
.
Create an Init Script
Set the Spring Boot application as a service to start on reboot:
- File: /etc/systemd/system/helloworld.service
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
[Unit] Description=Spring Boot HelloWorld After=syslog.target After=network.target[Service] User=username Type=simple [Service] ExecStart=/usr/bin/java -jar /home/linode/hello-world/build/libs/hello-world-0.0.1-SNAPSHOT.jar Restart=always StandardOutput=syslog StandardError=syslog SyslogIdentifier=helloworld [Install] WantedBy=multi-user.target
Start the service:
sudo systemctl start helloworld
Check the status is active:
sudo systemctl status helloworld
Reverse Proxy
Now that the Spring application is running as a service, an NGINX proxy allows opening the application to an unprivileged port and setting up SSL.
Create an NGINX configuration for the reverse proxy:
- File: /etc/nginx/conf.d/helloworld.conf
1 2 3 4 5 6 7 8 9 10 11 12 13
server { listen 80; listen [::]:80; server_name example.com; location / { proxy_pass http://localhost:8080/; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; proxy_set_header X-Forwarded-Proto $scheme; proxy_set_header X-Forwarded-Port $server_port; } }
Test the configuration to make sure there are no errors:
sudo nginx -t
If there are no errors, restart NGINX so the changes take effect:
sudo systemctl restart nginx
The application is now accessible through the browser. Navigate to the public IP address of the Linode and the “Hello world” message should appear.
More Information
You may wish to consult the following resources for additional information on this topic. While these are provided in the hope that they will be useful, please note that we cannot vouch for the accuracy or timeliness of externally hosted materials.
This page was originally published on